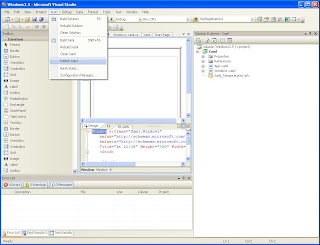
Saturday, March 21, 2009
Publishing/Deploying .NET 3.5 XAML applications
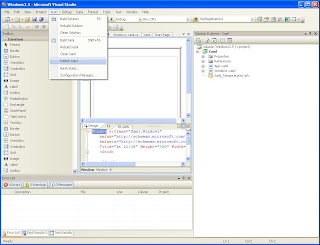
Sunday, January 11, 2009
Connecting to the Business Objects CMS with .NET
Tip:
Please note that the QueryBuilder SQL will return a maximum of 1000 records even if there are more to be returned. So logic should be included that if 1000 sorted records are returned, you should requery to retrieve missed records or until a recordset with less than 1000 returned records occurs.
This sample is a .NET 3.5 application.
Create a simple project and add references to
CrystalDecisions.Enterprise
CrystalDecisions.Enterprise.Framework
CrystalDecisions.Enterprise.InfoStore
Sample Code:
using System;
using CrystalDecisions.Enterprise;
namespace ConsoleAConnection
{
class Program
{
static void Main(string[] args)
{
string query = "Select SI_ID,SI_KIND,SI_NAME,SI_PARENT_FOLDER FROM CI_INFOOBJECTS WHERE SI_KIND IN ('Folder','FavoritesFolder')";
InfoObjects infoObjects = getCMCConnection().Query(query);
if (infoObjects.Count > 0)
{
foreach (InfoObject infoObject in infoObjects)
{
string siId = "" + infoObject.Properties["SI_ID"] + ":" + infoObject.Properties.Count;
Console.WriteLine("SI_ID=" + siId);
}
}
else
{
Console.WriteLine("No results found for " + query);
}
}
static InfoStore getCMCConnection()
{
SessionMgr sessionMgr = new SessionMgr();
EnterpriseSession enterpriseSession = sessionMgr.Logon("UserName", "Password", "ServerName", "secEnterprise");
EnterpriseService enterpriseService = enterpriseSession.GetService("InfoStore");
InfoStore infoStore = new InfoStore(enterpriseService);
return infoStore;
}
}
}
Thursday, January 8, 2009
Software Estimation by Steve McConnell
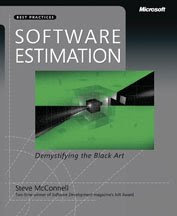
I just finished "Software Estimation" by Steve McConnell. This is a good book for covering exactly what the title indicates.
Steve McConnell is a well known author of software development books including "Code Complete".
This book starts with the difficulties experienced in software estimation and how hard it is indeed.
He then proceeds to cover approaches to develop more accurate estimates.
Techniques which include:
- counting
- calibration with historical data
- expert judgment
- decomposition and recomposition
- estimatino by analogy
- proxy-based estimates
Coverage of expert judgment in groups, software estimation tools, handling multiple approaches, and standardizing estimating procedures. The latter half of the book covers special issues in estimating.
All in all a good book to provide a thorough covering of software estimation.
Wednesday, January 7, 2009
ASP .NET 3.5 - Application Architecture and Design
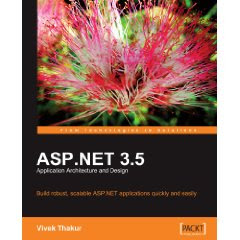
Overview of major issues in application architecture with a perspective in regards to .NET 3.5.
Chapter 1: Introduction to Architecture and Design
Sunday, December 14, 2008
Domain Driven Design by Eric Evans
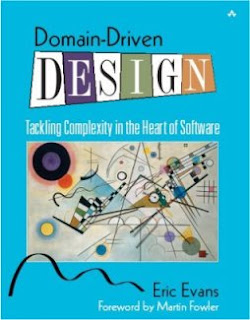
Premise of book
1. For most software projects, the primary focus should be on the domain and domain logic
2. Complex domain designs should be based on a model.
Part I - Putting the Domain Model to Work
Chapter 1 - Crunching Knowledge
Ingredients of Effective Modeling
1. Binding the model and the implementation - crude model type created and changed iteratively
2. Cultivating a language based on the model -explanations required between the software team and business
3. Developing a knowledge-rich model - captures knowledge of various kinds
4. Distilling the model - testing concepts and removing those which don't fit anymore
5. Brainstorming and experimenting
Chapter 2 - Communication and the Use of Language
When building your model use language that both sides(Development & Business) understand.
This is called "Ubiquitous language"
This helps make the model more useful for the conversation between development and business.
Chapter 3 - Binding Model and Implementation
A Domain Driven design calls for a model that doesn't just aid early analysis but is the very foundation for the design.
Tightly relating the code to an underlying model gives the code meaning and makes the model relevant.
Chapter 4 - Isolating the Domain
Give a Shipping Lane sample application with 4 tiers (User Interface, Application, Domain, and Infrastructure)
Layers can communicate down but not up.
Chapter 5 - A Model Expressed in Software
Introduce different types of objects
Entity Objects - objects defined by their identity(ie. key), can change, change state
Value Objects - objects that represent things that do not change, immutable (ie. an address)
3 attributes of a good service
1. operation relates to a domain concept that is not a natural part of an entity or value object
2. interface is defined in terms of other elements of the domain model
3. operation is stateless
Modules(aka Packages, namespaces)
grouping classes that are cohesive
Chapter 6 - The Life Cycle of a Domain Object
Talking about Aggregate Objects
Factories - Helping simpify the knowledge required to create complex objects
- each factory operation should be atomic
- the factory will be coupled to its arguments
Repositories
How to provide access to globally accessible objects
Repositories advantages include
- present clients with a simple model for obtaining persistent objects and managing their life cycle
- decouple application and domain design from persistence technolgoy, database,....
- communicate design decisions about object access
- allow easy substitution of a dummy implementation, for use in testing
Factory handles the beginning of an object's life; a Repository helps manage the middle and the end.
Chapter 7 - Using the Language: An Extended Example
An example of refinements in developing a Model-Driven Design via a Cargo Shipping System
Isolating the Domain, Distinguishing Entities and Value Objects, Aggregate Boundaries
Part III Refactoring Toward Deeper Insight
Chapter 8 - Breakthrough
Each refinement of code and model gives devlopers a clearer view.
This clarity creates the potential for a breakthrough of insights
Work on refactoring may not show much progress initially but often eventually leads to a breakthrough
Chapter 9 - Making Implicit Concepts Explicit
Listen to the language the domain experts use. These are hints of a concept that might benefit the model.
Scrutinize Awkwardness in language describing the model.
There may be a missing concept
Descibed patterns such as Specification - testing objects for certain criteria
Chapter 10 - Supple Design
Intention-Revealing Interfaces - better expression
Side-Effect-Free Functions - make safe and simple
Assertions - make side effects explicit
Standalone classes - Lower coupling, elimnate all other concepts from the picture
Closure of Operations - where it fits, define an operation whose return typ is the same as the type of its arguments
- this provides a high-level interface without introductin any dependency on other concepts
Conceptual Contours - decompose design elements into cohesive units, taking into consideration your intuation
Chapter 11- Applying Analysis Patterns
Analysis patterns are groups of concepts that represent a common construction in business modelling.
It may be releveant to only one domain or it may span many domains. [Fower 1997, p.8]
-they help feed into the dynamo of knowledge crunching and refactoring toward deeper insight and stimulates development
-when you use a term from a well-known analysis pattern, take care to keep the basic concept it designates intact
Chapter 12 - Relating Design Patterns to the Model
Strategy - decoupling an algorithm via an interface which allows other algorithms to be interchanged
Composite - treating individual objects and compositions of objects uniformly
Chapter 13 - Refactoring Toward Deeper Insight
Focus on:
1. Live in the domain
2. Keep looking at things a different way
3. Maintain an unbroken dialog with domain experts
Part IV - Strategic Design
Chapter 14 - Maintaining Model Integrity
Bounded Context - explicitly define the context within wich a model applies
Continuous Integration - institute a process of merging all code and other implementation artifacts frequently
Context Map - identify each model in play on the project and define its BOUNDED CONTEXT
Shared Kernel - designate some subset of the domain model that the 2 teams agree to share
Customer/Supplier Development Teams - establish a clear customer/supplier relationship between two teams
Conformist - if there is an upstream/downstream relationship, the downstream should conform to the upstream group
Anticorruption Layer - create an isolating layer to provide clients with functionality in terms of their own domain model.
Separate Ways - declare a bounded context to have no connection to others at all(avoid cost of integration)
Open Host Service - define a protocol that gives access to your subsystem as a set of Services
Published Language - use a well-documented shared language that can express the necessary domain infomration as a common medium of communication
Chapter 15 - Distillation
Core Domain - Boil the model down. Put most valuable and specialized concepts into the Core Domain, apply top talent to the Core Domain
Generic Subdomains - identify cohesive subdomains that are not motivation for your project, leave no trace of project specifics there
Domain Vision Statement - write short description of the Core domain ("value proposition"), statement to guide
Highlighted Core - very brief document (3-7 pgs) that describes the core domain
Cohesive Mechanisms - partition a conceptually cohesive mechanism into a separate lightweight framework(use intention revealing interface)
Segregated Core - refactore the model to separate the core concepts from supporting players and strengthen the cohesion of the CORE while reducing its coupling to other code
Abstract Core - Identify the most fundamental concepts in the model and factor them into distinct classes, abstract classes, or interfaces, place in own module
Chapter 16 - Large Scale Structure
Devising a pattern of rules or roles and relationshiops that will span the entire system and that allows some understanding of each part's place in the whole
Evolving Order - the conceptual large-scale structure evovolving with the application, possibly changing to a completely different type of structure along the way, avoid straight-jacketing
System Metaphor - when a concrete analogy to the system emerges that captures the imagination of team members and seems to lead thinking in a useful direction, adopt it as a large-scale structure
Responsibility Layers - look at conceptual dependencies in your model and the varying rates and sources of change of different parts of your domain, if you identify a natural strata in the domain, cast them as broad abstract responsibilities
Knowledge Level - a group of objects that describes how another group of objects should behave, crate a distinct set of objects that can be used to describe and constrain the structure and behavior of the basic model
Pluggable Component Framework - distill an abstract core of interfaces and interactions and create a framework that allows diverse implementations of those interfaces to be freely substituted
Chapter 17 - Bringing the Strategy Together
Six essentials for Strategic Design Decision Making
1. Decisions must reach the entire team
2. The decision process must absorbe feedback
3. The plan must allow for evolution
4. Architecture teams must not siphon off all the best and brightest
5. Strategic design requires minimalism and humility
6. Objects are specialists; developers are generalists
Thursday, November 20, 2008
Dynamic Checkerboard Code
The following java solution is proposed
/*
* Create a function that can draw a checkerboard with boardwidth by boardlength where each square is squarewidth by squarelength.
* It is to be drawn with System.out.prints only.
*/
public class DrawCheckerBoard {
public static void drawCheckerBoard(int checkerBoardWidth, int checkerBoardHeight, int squareWidth, int squareHeight)
{
for (int i = 0 ; i < checkerBoardHeight ; i++)
{
for (int j = 0 ; j < squareHeight ; j++)
{
for(int k = 0 ; k < checkerBoardWidth ; k++)
{
//determine character to draw for square
//based on the which checkerboard spot (i and k indices)
String character = "X";
if ((i+k)%2==0)
{
character = "O";
}
for (int l = 0 ; l < squareWidth ; l++)
{
System.out.print(character);
}
}//end of line
System.out.println("");
}
}
}
public static void main(String[] args)
{
System.out.println("4 by 4 board with each square being 2 by 2");
drawCheckerBoard(4,4,2,2);
System.out.println("8 by 8 board with each square being 4 by 4");
drawCheckerBoard(8,8,4,4);
}
}
Monday, September 22, 2008
COBIT Powerpoint Presentation
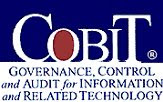
I researched to find out what COBIT was by reading IT Governance Based on Cobit 4.1: A Management Guide (Paperback)
by Koen Brand and Harry Boonen.
COBIT is an IT Framework for Governance. It has similarities to ITIL and some overlap. One person told me that COBIT is what you build and ITIL is how you do it.
Anyways, I made a brief powerpoint presentation on COBIT which may be viewed from Here
Obviously very high level, but with pictures and some text, it will help.